Integrating AWS Secrets Manager With Spring Boot
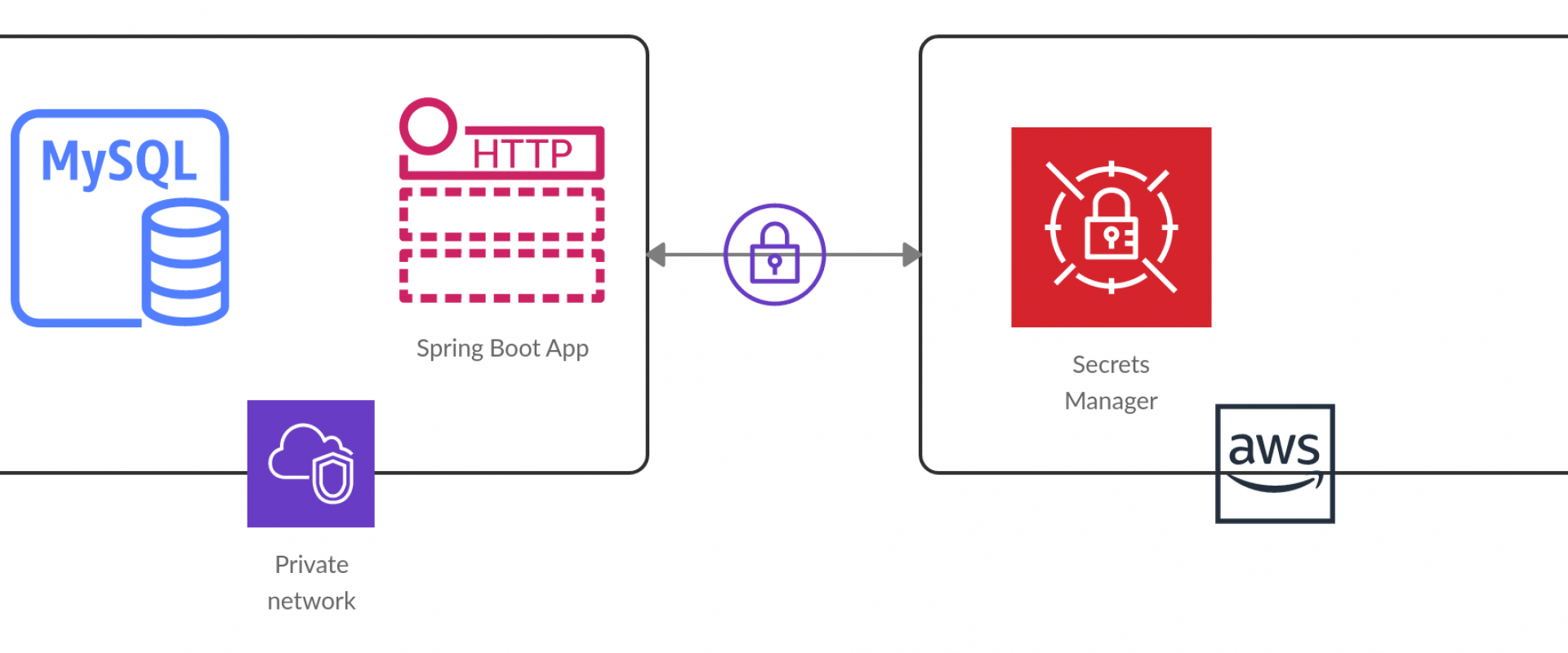
In this article, readers learn how Amazon Secrets Manager is a powerful tool that helps developers manage application secrets more securely with Spring Boot.
In a microservices architecture, it’s common to have multiple services that need access to sensitive information, such as API keys, passwords, or certificates. Storing this sensitive information in code or configuration files is not secure because it’s easy for attackers to gain access to this information if they can access your source code or configuration files.
To protect sensitive information, microservices often use a secrets management system, such as Amazon Secrets Manager, to securely store and manage this information. Secrets management systems provide a secure and centralized way to store and manage secrets, and they typically provide features such as encryption, access control, and auditing.
Amazon Secrets Manager is a fully managed service that makes it easy to store and retrieve secrets, such as database credentials, API keys, and other sensitive information. It provides a secure and scalable way to store secrets, and integrates with other AWS services to enable secure access to these secrets from your applications and services.
Some benefits of using Amazon Secrets Manager in your microservices include:
• Centralized management: You can store all your secrets in a central location, which makes it easier to manage and rotate them.
• Fine-grained access control: You can control who has access to your secrets, and use AWS Identity and Access Management (IAM) policies to grant or revoke access as needed.
• Automatic rotation: You can configure Amazon Secrets Manager to automatically rotate your secrets on a schedule, which reduces the risk of compromised secrets.
• Integration with other AWS services: You can use Amazon Secrets Manager to securely access secrets from other AWS services, such as Amazon RDS or AWS Lambda.
Overall, using a secrets management system, like Amazon Secrets Manager, can help improve the security of your microservices by reducing the risk of sensitive information being exposed or compromised.
In this article, we will discuss how you can define a secret in Amazon Secrets Manager and later pull it using the Spring Boot microservice.
Creating the Secret
To create a new secret in Amazon Secrets Manager, you can follow these steps:
1. Open the Amazon Secrets Manager console by navigating to the “AWS Management Console,” selecting “Secrets Manager” from the list of services, and then clicking “Create secret” on the main page.
2. Choose the type of secret you want to create: You can choose between “Credentials for RDS database” or “Other type of secrets.” If you select “Other type of secrets,” you will need to enter a custom name for your secret.
3. Enter the secret details: The information you need to enter will depend on the type of secret you are creating. For example, if you are creating a database credential, you will need to enter the username and password for the database.
4. Configure the encryption settings: By default, Amazon Secrets Manager uses AWS KMS to encrypt your secrets. You can choose to use the default KMS key or select a custom key.
5. Define the secret permissions: You can define who can access the secret by adding one or more AWS Identity and Access Management (IAM) policies.
6. Review and create the secret: Once you have entered all the required information, review your settings and click “Create secret” to create the secret.
Alternatively, you can also create secrets programmatically using AWS SDK or CLI. Here’s an example of how you can create a new secret using the AWS CLI:
Shell
aws secretsmanager create-secret --name my-secret --secret-string '{"username": "myuser", "password": "mypassword"}'
This command creates a new secret called “my-secret” with a JSON-formatted secret string containing a username and password. You can replace the secret string with any other JSON-formatted data you want to store as a secret.
You can also create these secrets from your microservice as well:
1. Add the AWS SDK for Java dependency to your project: You can do this by adding the following dependency to your pom.xml file:
XML
2. Initialize the AWS Secrets Manager client: You can do this by adding the following code to your Spring Boot application’s configuration class:
Java
@Configuration
public class AwsConfig {
@Value("${aws.region}")
private String awsRegion;
@Bean
public AWSSecretsManager awsSecretsManager() {
return AWSSecretsManagerClientBuilder.standard()
.withRegion(awsRegion)
.build();
}
}
This code creates a new bean for the AWS Secrets Manager client and injects the AWS region from the application.properties file.
3. Create a new secret: You can do this by adding the following code to your Spring Boot service class:
Java
@Autowired
private AWSSecretsManager awsSecretsManager;
Public void createSecret(String secretName, String secretValue) {
CreateSecretRequest request = new CreateSecretRequest()
.withName(secretName)
.withSecretString(secretValue);
CreateSecretResult result = awsSecretsManager.createSecret(request);
String arn = result.getARN();
System.out.println("Created secret with ARN: " + arn);
}
This code creates a new secret with the specified name and value. It uses the CreateSecretRequest class to specify the name and value of the secret and then calls the createSecret method of the AWS Secrets Manager client to create the secret. The method returns a CreateSecretResult object, which contains the ARN (Amazon Resource Name) of the newly created secret.
These are just some basic steps to create secrets in Amazon Secrets Manager. Depending on your use case and requirements, there may be additional configuration or setup needed.
Pulling the Secret Using Microservices
Here are the complete steps for pulling a secret from the Amazon Secrets Manager using Spring Boot:
1. First, you need to add the following dependencies to your Spring Boot project:
XML
com.amazonaws
aws-java-sdk-secretsmanager
1.12.37
com.amazonaws
aws-java-sdk-core
1.12.37
org.springframework.cloud
spring-cloud-starter-aws
2.3.2.RELEASE
2. Next, you need to configure the AWS credentials and region in your application.yml file:
YAML
aws:
accessKey:
secretKey:
region:
3. Create a configuration class for pulling the secret:
Java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.cloud.aws.secretsmanager.AwsSecretsManagerPropertySource;
import org.springframework.context.annotation.Configuration;
import com.amazonaws.services.secretsmanager.AWSSecretsManager;
import com.amazonaws.services.secretsmanager.AWSSecretsManagerClientBuilder;
import com.amazonaws.services.secretsmanager.model.GetSecretValueRequest;
import com.amazonaws.services.secretsmanager.model.GetSecretValueResult;
import com.fasterxml.jackson.databind.ObjectMapper;
@Configuration
public class SecretsManagerPullConfig {
@Autowired
private AwsSecretsManagerPropertySource awsSecretsManagerPropertySource;
public T getSecret(String secretName, Class valueType) throws Exception {
AWSSecretsManager client = AWSSecretsManagerClientBuilder.defaultClient();
String secretId = awsSecretsManagerPropertySource.getProperty(secretName);
GetSecretValueRequest getSecretValueRequest = new GetSecretValueRequest()
.withSecretId(secretId);
GetSecretValueResult getSecretValueResult = client.getSecretValue(getSecretValueRequest);
String secretString = getSecretValueResult.getSecretString();
ObjectMapper objectMapper = new ObjectMapper();
return objectMapper.readValue(secretString, valueType);
}
}
4. In your Spring Boot service, you can inject the SecretsManagerPullConfig class and call the getSecret method to retrieve the secret:
Java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class MyService {
@Autowired
private SecretsManagerPullConfig secretsManagerPullConfig;
public void myMethod() throws Exception {
MySecrets mySecrets = secretsManagerPullConfig.getSecret("mySecrets", MySecrets.class);
System.out.println(mySecrets.getUsername());
System.out.println(mySecrets.getPassword());
}
}
In the above example, MySecrets is a Java class that represents the structure of the secret in the Amazon Secrets Manager. The getSecret method returns an instance of MySecrets that contains the values of the secret.
Note: The above code assumes the Spring Boot application is running on an EC2 instance with an IAM role that has permission to read the secret from the Amazon Secrets Manager. If you are running the application locally or on a different environment, you will need to provide AWS credentials with the necessary permissions to read the secret.
Conclusion
Amazon Secrets Manager is a secure and convenient way to store and manage secrets such as API keys, database credentials, and other sensitive information in the cloud. By using Amazon Secrets Manager, you can avoid hardcoding secrets in your Spring Boot application and, instead, retrieve them securely at runtime. This reduces the risk of exposing sensitive data in your code and makes it easier to manage secrets across different environments.
Integrating Amazon Secrets Manager with Spring Boot is a straightforward process thanks to AWS SDK for Java. With just a few lines of code, you can create and retrieve secrets from Amazon Secrets Manager in your Spring Boot application. This allows you to build more secure and scalable applications that can be easily deployed to the cloud.
Overall, Amazon Secrets Manager is a powerful tool that can help you manage your application secrets in a more secure and efficient way. By integrating it with Spring Boot, you can take advantage of its features and benefits without compromising on the performance or functionality of your application.
We Provide consulting, implementation, and management services on DevOps, DevSecOps, Cloud, Automated Ops, Microservices, Infrastructure, and Security
Services offered by us: https://www.zippyops.com/services
Our Products: https://www.zippyops.com/products
Our Solutions: https://www.zippyops.com/solutions
For Demo, videos check out YouTube Playlist: https://www.youtube.com/watch?v=4FYvPooN_Tg&list=PLCJ3JpanNyCfXlHahZhYgJH9-rV6ouPro
If this seems interesting, please email us at [email protected] for a call.
Relevant Blogs:
How to Choose the Right Data Masking Tool for Your Organization
Recent Comments
No comments
Leave a Comment
We will be happy to hear what you think about this post