Zabbix custom scripts
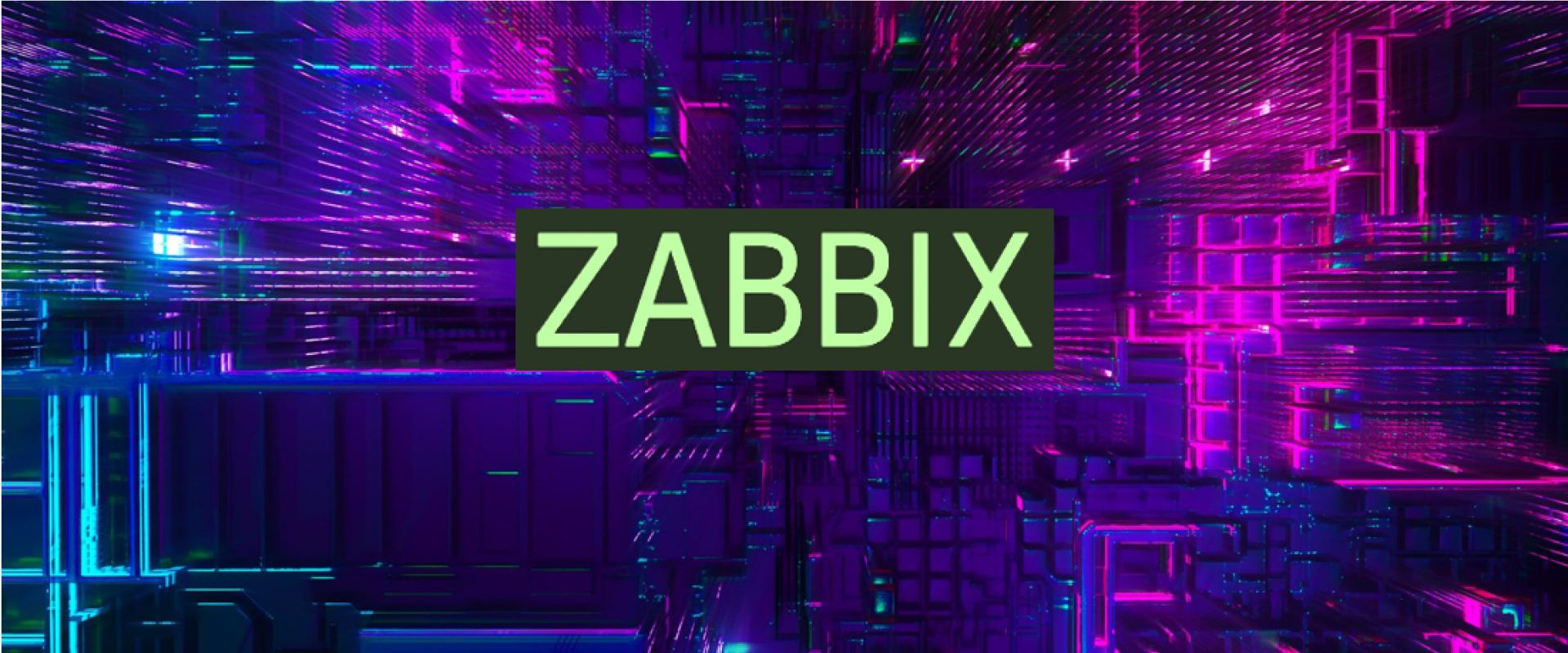
If you want to monitor custom parameters on Zabbix, you can write a script to monitor and share the parameter to the Zabbix server.
Below is an example, where we are creating a custom python script to report service status in linux.
Create a folder on our host
#mkdir /home/zabbix
#cd /home/zabbix
And create a new Python file
# cat service_discovery.py
#!/usr/bin/python
import sys
import os
import json
SERVICES = os.popen('systemctl list-unit-files').read()
ILLEGAL_CHARS = ["\\", "'", "\"", "`", "*", "?", "[", "]", "{", "}", "~", "$", "!", "&", ";", "(", ")", "<", ">", "|", "#", "@", "0x0a"]
DISCOVERY_LIST = {"data": []}
LINES = SERVICES.splitlines()
for l in LINES:
service_unit = l.split(".service")
if len(service_unit) == 2:
if not [ele for ele in ILLEGAL_CHARS if(ele in service_unit[0])]:
status = service_unit[1].split()
if len(status) == 1:
if (status[0] == "enabled" or status[0] == "generated"):
DISCOVERY_LIST["data"].append({"{#SERVICE}": service_unit[0]})
else:
if (status[0] == "enabled" or status[0] == "generated") and status[1] == "enabled":
DISCOVERY_LIST["data"].append({"{#SERVICE}": service_unit[0]})
JSON = json.dumps(DISCOVERY_LIST)
print(JSON)
Need to give execute permissions
#sudo chmod a+x service_discovery.py
we can the test it works from the Zabbix users perspective by running
#sudo -H -u zabbix bash -c '/home/zabbix/service_discovery.py'
Create a UserParameter in the Zabbix agent conf.
Scroll down to the UserParameters section and add these new UserParameters
UserParameter=service.discovery,/home/zabbix/service_discovery.py
UserParameter=service.isactive[*],systemctl is-active --quiet '$1' && echo 1 || echo 0
UserParameter=service.activatedtime[*],systemctl show '$1' --property=ActiveEnterTimestampMonotonic | cut -d= -f2
Restart Zabbix Agent and check status
#sudo service zabbix-agent restart
#sudo service zabbix-agent status
Test the service.discovery UserParameter using
# zabbix_agentd -t service.discovery
service.discovery [t|{"data": [{"{#SERVICE}": "auditd"}, {"{#SERVICE}": "chronyd"}, {"{#SERVICE}": "cloud-config"}, {"{#SERVICE}": "cloud-final"}, {"{#SERVICE}": "cloud-init-local"}, {"{#SERVICE}": "cloud-init"}, {"{#SERVICE}": "crond"}, {"{#SERVICE}": "dbus-org.fedoraproject.FirewallD1"}, {"{#SERVICE}": "firewalld"}, {"{#SERVICE}": "ipmi"}, {"{#SERVICE}": "irqbalance"}, {"{#SERVICE}": "kdump"}, {"{#SERVICE}": "microcode"}, {"{#SERVICE}": "mysqld"}, {"{#SERVICE}": "postfix"}, {"{#SERVICE}": "qemu-guest-agent"}, {"{#SERVICE}": "rhel-autorelabel-mark"}, {"{#SERVICE}": "rhel-autorelabel"}, {"{#SERVICE}": "rhel-configure"}, {"{#SERVICE}": "rhel-dmesg"}, {"{#SERVICE}": "rhel-domainname"}, {"{#SERVICE}": "rhel-import-state"}, {"{#SERVICE}": "rhel-loadmodules"}, {"{#SERVICE}": "rhel-readonly"}, {"{#SERVICE}": "rpcbind"}, {"{#SERVICE}": "rsyslog"}, {"{#SERVICE}": "sshd"}, {"{#SERVICE}": "systemd-readahead-collect"}, {"{#SERVICE}": "systemd-readahead-drop"}, {"{#SERVICE}": "systemd-readahead-replay"}, {"{#SERVICE}": "tuned"}, {"{#SERVICE}": "zabbix-agent"}, {"{#SERVICE}": "zabbix-server"}]}]
The response should resemble this below, but containing the various available services depending on your host.
Relevant Blogs:
Recent Comments
No comments
Leave a Comment
We will be happy to hear what you think about this post